Print Odd Numbers In Java
Hi Readers, In this post, we are going to write a core Java Program to print Odd numbers within a given limit.
Java Program to print Odd and Even Numbers from an Array. We can print odd and even numbers from an array in java by getting remainder of each element and checking if it is divided by 2 or not. If it is divided by 2, it is even number otherwise it is odd number. Output: Odd Numbers: 1 5 3 Even Numbers: 2 6 2. ← prev next →.
- Java Program to Print Odd Numbers from 1 to N Example 1. This program allows user to enter the maximum limit value, and prints the odd numbers from 1 to maximum limit value using For Loop and If statement. In Java we have% (Module) Arithmetic Operator to check the remainder. If the remainder is not 0 then the number is odd.
- Java Program to Print Odd Numbers from 1 to N Example 1. This program allows user to enter the maximum limit value, and prints the odd numbers from 1 to maximum limit value using For Loop and If statement.
First of all, you should know how to find an Odd number and it is pretty simple, divide any number by 2 if the remainder is not zero then it’s an Odd number.
So let’s see the complete Java Program here:
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 | * */ Scanner in=newScanner(System.in); //get the limit from user limit=in.nextInt(); //print the odd numbers for(i=1;i<=limit;i++){ //divide by 2 if the remainder is not 0 then it is an odd number System.out.println(i); } |
Read the inline comments to understand the logic.
The output of the above program:
Below is the code I'm stuck with.
user1803551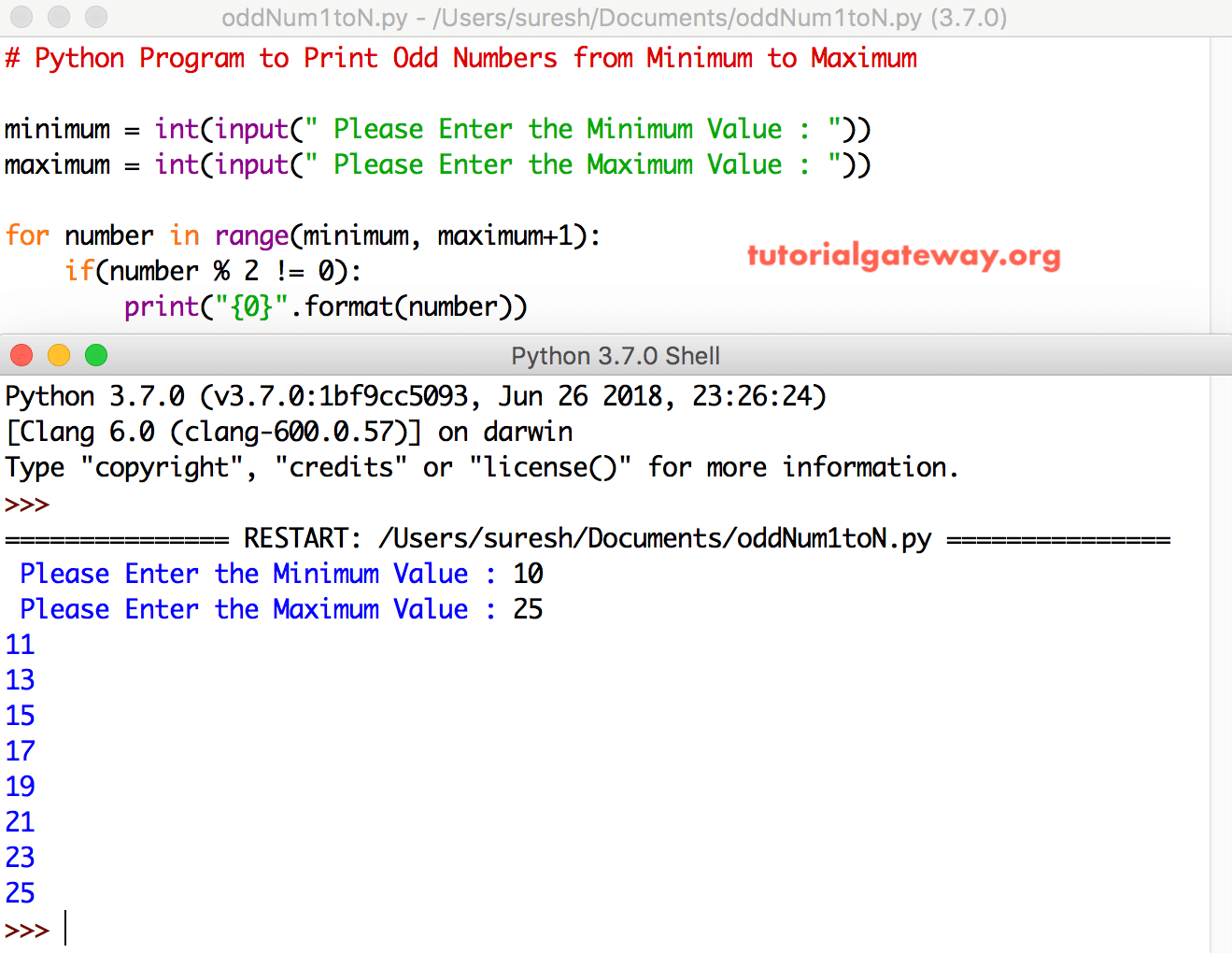
8 Answers
first you need to calculate your number, try
but this problem you can solve with single loop
brso05This code will allow you to do what you want with 1 loop which is more efficient than using nested loops.
This will loop through each number from 1-99 and print if it is odd. If the number is a multiple of 10 then it will print a new line.
brso05brso05Maybe this helps:
Other alternative with just one loop:
Print Odd Numbers In Array Java
If you want to use a nested forloop you have to use both iterating variables to build your numbers. You dont use i at all in the inner for-loop. Therefore only 1 3 5 7 9 gets printed 9 times. For a hotfix try to use something like
instead of note how i+j does not compute an addition here.Anyway like pointed out in the comments you dont really need 2 loops here.we cheat:
we check:
both output same, 5 odd numbers in a row, without trailing spaces.
Kent
With this one you can easily set the number of columns as you like it.
We use a single loop. Rome total war seleucid units.

Code:
Output:
However, if I understood your question correctly, you wanted to show numbers with 5 columns and 9 rows? Well this is impossible if we print only the numbers from 1 to 99. With 5 columns and numbers 1 to 99, you will get 10 rows.
Olavi MustanojaOlavi MustanojaYour code now prints 9 rows of:
This is happening because your inner loop loops on values between 1 and 9, always.
You should try something like:
This will print:
MarounMarounNot the answer you're looking for? Browse other questions tagged javaloopsfor-loopnested or ask your own question.
Comments are closed.